CSS color
property is used to set the color of text in a webpage. For example,
h1 {
color: blue;
}
Browser Output
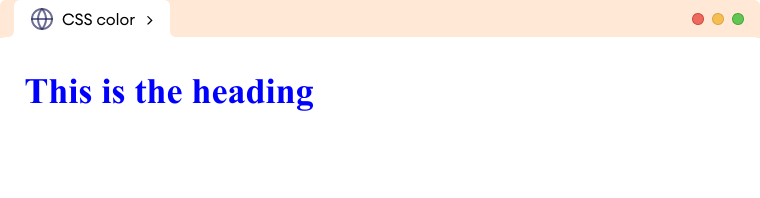
Here, color: blue
sets the color of h1
element to blue
.
CSS Color Types
In CSS, the value for color
property can be specified by the following color formats:
- Named colors:
red
,blue
,green
, etc - Hex colors:
#FF0033
,#F03
, etc - RGB and RGBa colors:
rgb(0, 0, 255)
,rgba(0, 0, 255, 0.5)
, etc - HSL and HLSa colors:
hsl(180, 100%, 75%)
,hlsa(180, 100%, 75%, 0.5)
, etc
Note: The color
property is also known as foreground color.
CSS Named Colors
CSS named colors refers to the set of predefined colors names that can be used in our CSS. Named colors are easy to remember and use. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>Named colors</title>
</head>
<body>
<h1>CSS Color Property</h1>
</body>
</html>
h1 {
/* sets the color of h1 element to blue */
color: blue;
}
Browser Output
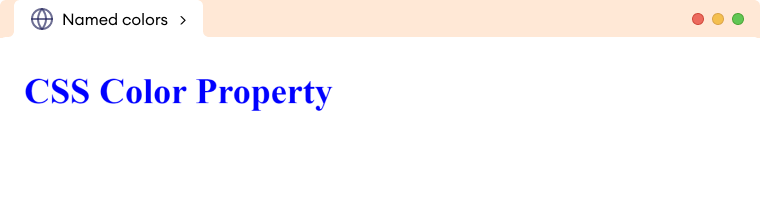
There are around 147 colors names recognized by browsers like cyan
, orange
, red
, pink
, crimson
and so on.
CSS RGB Colors
The RGB
colors are based on the combination of three primary colors: red
, green
and blue
. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>RGB Color format</title>
</head>
<body>
<h1 class="rgb_red_value">RGB(255, 0, 0) represents red</h1>
<h1 class="rgb_red_percentage">RGB(100%, 0, 0) represents red</h1>
<h1 class="rgb_green_value">RGB(0, 255, 0) represents green</h1>
<h1 class="rgb_blue_value">RGB(0, 0, 255) represents blue</h1>
<h1 class="rgb_black_value">RGB(0, 0, 0) represents black</h1>
</body>
</html>
h1.rgb_red_value {
/* sets the color of h1 element to red */
color: rgb(255, 0, 0);
}
h1.rgb_red_percentage {
/* sets the color of h1 element to red */
color: rgb(255, 0, 0);
}
h1.rgb_green_value {
/* sets the color of h1 element to green */
color: rgb(0, 255, 0);
}
h1.rgb_blue_value {
/* sets the color of h1 element to blue */
color: rgb(0, 0, 255);
}
h1.rgb_black_value {
/* sets the color of h1 element to black */
color: rgb(0, 0, 0);
}
Browser output
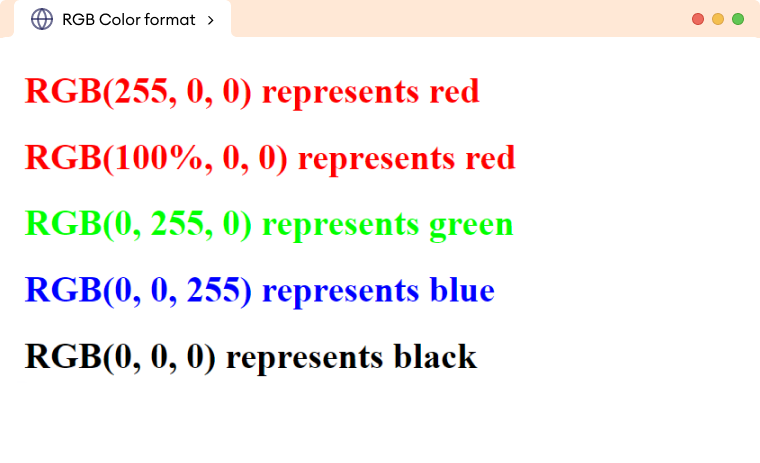
In the above code, rgb(255, 0, 0)
the numeric values represent the intensity of red
, green
, and blue
color respectively.
The RGB
colors are specified with a comma-separated list of three numeric values either ranging from 0 to 255 or percentage values ranging from 0% to 100%. For example, the rgb(255, 0, 0)
is equivalent to rgb(100%, 0, 0)
.
Note: The combination of rgb
values can generate millions of different colors.
CSS RGBa Colors
The RGBa
colors work the same as RGB
colors except we have a new parameter a
which stands for alpha. The value of alpha sets the opacity of the color. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>RGBa Color Format</title>
</head>
<body>
<h1>rgba(255, 0, 0, 0.5) represents red with opacity 0.5</h1>
</body>
</html>
h1 {
/* sets the color of h1 element to red with 50% opacity*/
color: rgba(255, 0, 0, 0.5);
}
Browser Output
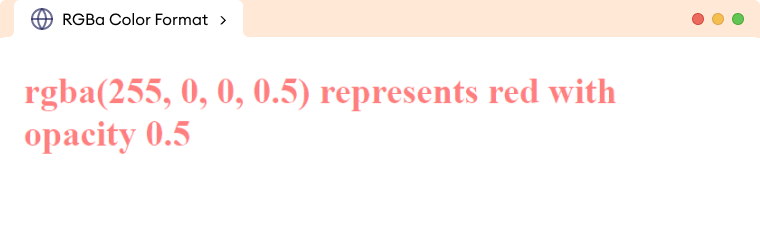
In the above code, rgb(255, 0, 0, 0.5)
the numeric values represent the intensity of red
, green
, blue
and opacity
respectively.
The RGBa
colors are specified with a comma-separated list of four numeric values. The left three values range from 0 to 255 or percentage values range from 0% to 100%. And the final value ranges from 0 (fully transparent) to 1(fully opaque) representing the intensity of opacity.
CSS Hexadecimal Colors
Hexadecimal colors, which are also known as hex values, are represented by six digits of hexadecimal code. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>Hexadecimal colors</title>
</head>
<body>
<h1 class="red_hex_value">#ff0000 represents red</h1>
<h1 class="red_short_hex_value">#f00 represents red</h1>
<h1 class="green_hex_value">#00ff00 represents green</h1>
<h1 class="blue_hex_value">#0000ff represents blue</h1>
<h1 class="black_hex_value">#000000 represents black</h1>
</body>
</html>
h1.red_hex_value {
/* sets the color of h1 element to red*/
color: #ff0000;
}
h1.red_short_hex_value {
/* sets the color of h1 element to red*/
color: #f00;
}
h1.green_hex_value {
/* sets the color of h1 element to green*/
color: #00ff00; /*equivalent to #0f0 */
}
h1.blue_hex_value {
/* sets the color of h1 element to blue*/
color: #0000ff; /*equivalent to #00f*/
}
h1.black_hex_value {
/* sets the color of h1 element to black*/
color: #000000; /*equivalent to #000*/
}
Browser output
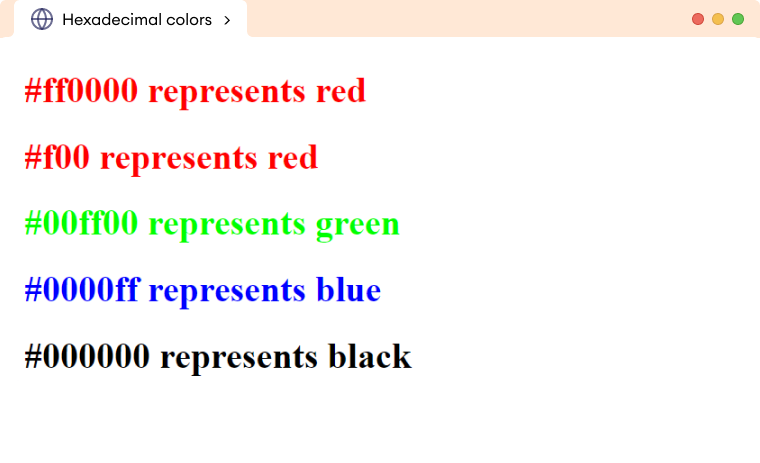
In the above code, #ff0000
the pair of numeric values from the left represent the intensity of red
, green
, and blue
colors respectively.
The six digits in hex
colors can be any number from 0 to 9 or any letter from A
to F
, and together they define a specific color.
Note:If two consecutive digits of all hex color codes are the same, we can use only one digit for it. For example, #ff0000
can be shortened to #f00
which still represents the red
color.
CSS HSL Colors
HSL
colors are the combination of three components of hue, saturation, and lightness. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>HSL color format</title>
</head>
<body>
<h1> hsl(0, 100%, 50%) represents red</h1>
</body>
</html>
h1 {
color: hsl(0, 100%, 50%);
}
Browser Output
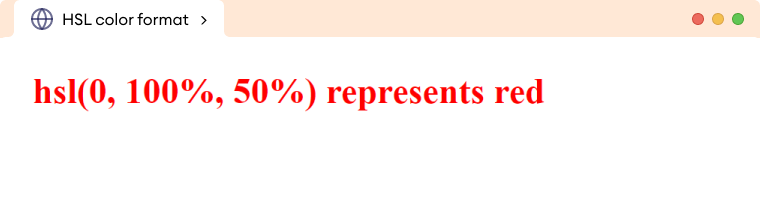
In the above code, hsl(0, 100%, 50%)
,
- The first value 0 represents
hue
and takes a numeric value ranging from 0 to 360. The value 0 representsred
, 120 representsgreen
and 240 representsblue
. - The second value
100%
representssaturation
which refers to the intensity ofhue
. The value ranges from0%
(fullygray
) to100%
(fully saturated). - The third value represents
lightness
which refers to the brightness of the color. The value ranges from0%
(fullyblack
) to100%
(fullywhite
).
CSS HSLa Colors
The HLSa
colors work the same as HSL
colors except we have a new parameter a
which stands for alpha. The value of alpha sets the opacity of the color. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>HLSa Color format</title>
</head>
<body>
<h1>hsla(0, 100%, 50%, 0.4) represents light red</h1>
</body>
</html>
h1 {
color: hsla(0, 100%, 50%, 0.4);
}
Browser output
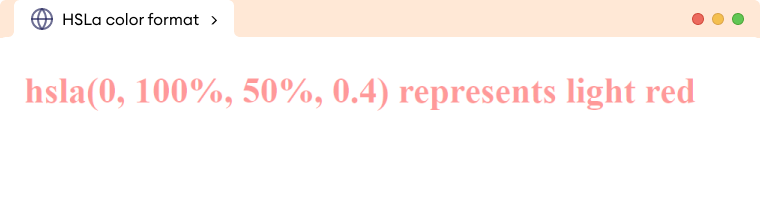
In the above code, hsla(0, 100%, 50%, 0.4)
, the left first three values represent hue
, saturation
and lightness
the same as hsl
colors. And the, final value 0.4
represents the opacity whose value ranges from 0(fully transparent) to 1(fully opaque).
Significance of Contrast
Sufficient contrast between the text color and background color helps individuals with visual impairments or color blindness to read with ease. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>Contrast Importance</title>
</head>
<body>
<h1>Good Contrast for Readability</h1>
<p class="good_contrast">
This example provides sufficient contrast between the two colors,
making the text easy to read. This provides sufficient contrast
between the two colors, making the text easy to read.
</p>
<h1>Poor Contrast for Readability</h1>
<p class="poor_contrast">
This text is difficult to read for the individuals with visual
impairments or color blindness. So it is important to ensure
sufficient contrast between the text color and background color is
available.
</p>
</body>
</html>
p.good_contrast {
color: #333;
background-color: #f2f2f2;
}
p.poor_contrast {
color: #98a6a7;
background-color: #c7d3d4;
}
Browser Output
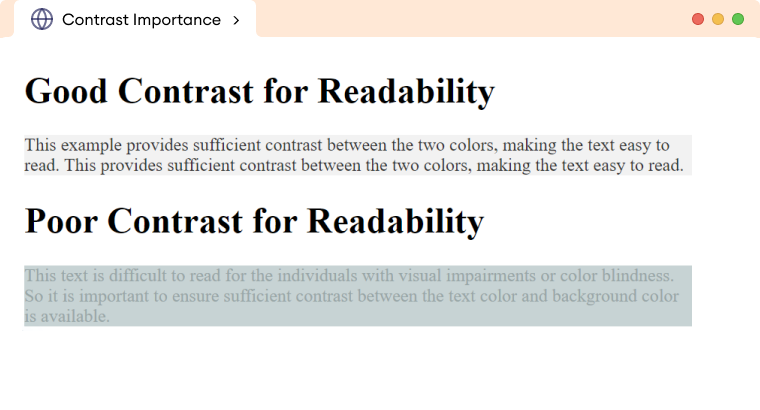